In Java Object-Oriented Programming (OOP), the "static" keyword significantly shapes how data and behaviour are shared among objects and classes. Let's explore this concept using a "Person" class with a simple example.
1. The "Person" Class
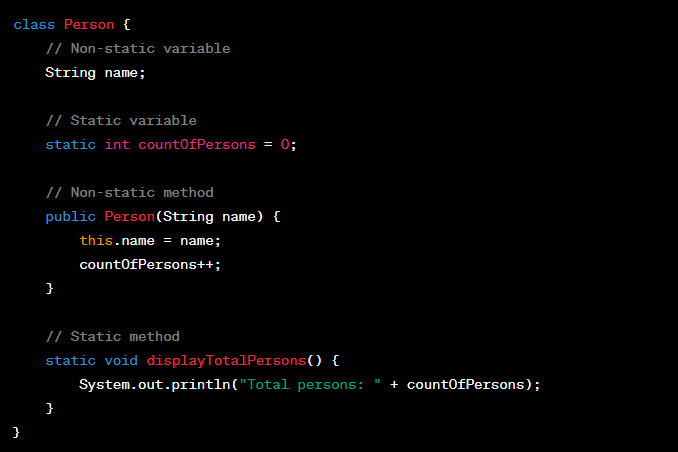
1.1 Non-static Elements
Non-static Variable ("name"): Each "Person" object has its own "name" attribute, representing the unique name of that person.
Non-static Method ("Person(String name)"): The constructor initializes the non-static variables when an object is created. It also increments the "countOfPersons", reflecting the total number of "Person" objects.
1.2 Static Elements
Static Variable ("countOfPersons"): This variable is shared among all instances of the "Person" class. It keeps track of the total number of "Person" objects created, regardless of the specific instance.
Static Method ("displayTotalPersons()"): This method is not tied to a particular instance but belongs to the class itself. It can be called without creating a "Person" object and displays the total number of persons.
2. Inheritance with "Student" and "Teacher"
Now, let's extend the "Person" class with "Student" and "Teacher" classes:
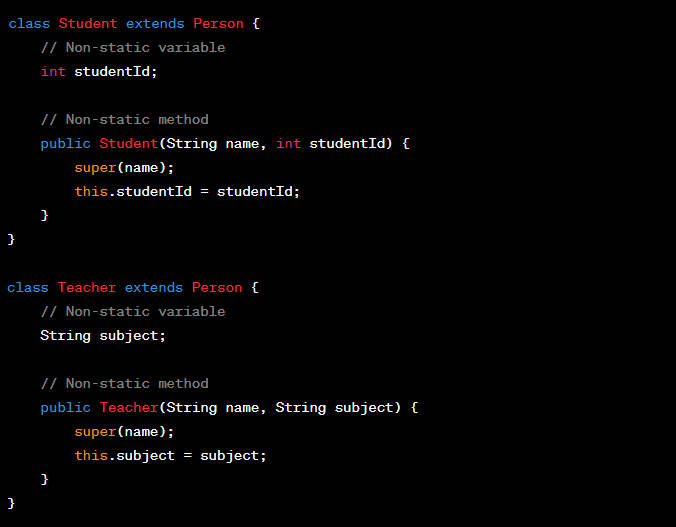
Both "Student" and "Teacher" inherit non-static elements from the "Person" class, showcasing the concept of code reuse and hierarchy in OOP.
3. Putting It All Together
In the Main class, we create instances of "Person", "Student", and "Teacher". The static method "displayTotalPersons()" demonstrates how static elements are accessed without the need for object instantiation and it will return 3.
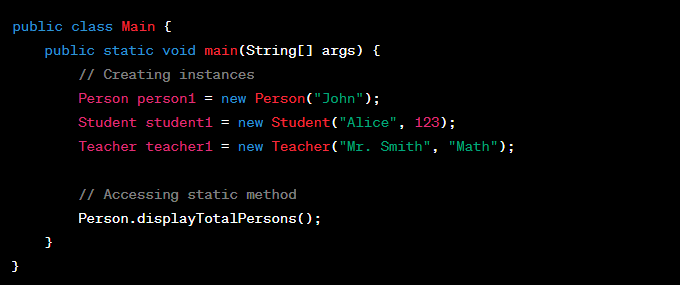
4. Conclusion
Understanding the "static" keyword in Java OOP is crucial for efficient code organization and resource management. It allows for the creation of shared data and behaviour at the class level, providing flexibility and control in designing robust and maintainable object-oriented systems.
Comments